On some occasions , we would like to a point in unity world space and find it’s equivalent in chart coordinates. On some occasions we would also like to do the opposite , and to take a point in chart coordinates and find it’s equivalent in world space coordinates. Graph and Chart contains methods that do this conversion for you. This article covers methods to convert between chart space and world space.
After reading this article it is recommended to view the marker tutorials that are located in Tutorials/Marker in the Graph and Chart folder. It is also recommended to view Placing your own objects on top of charts for a use case example of the methods described in this article
Notice: This articles assumes you have already learned about the different charts of Graph and Chart in the previous tutorials
What are Chart Coordinates
Chart coordinates are the coordinates you specify when adding data to the chart. For example , when you call AddPointToCategory on the graph chart , you specify the point data:
graph.DataSource.AddPointToCategory("Player 1",5,5);
The above line , places a point on the chart coordinate of 5,5;
If you are using date time with your chart you need to use ChartDateUtillity, make sure to look at Date, Time and Format to understand how to apply dates and times into the following methods.
Graph Chart And Candle Chart
The following methods allow you to convert between chart space and world space on the Graph and Candle chart:
1. PointToWorldSpace
this method gets a point in chart coordinates and converts it to a point in world space. For canvas charts , it will convert it to the coordinates inside the canvas in which the chart is in. On some occasions the point specified is not shown on the view portion , in this cases the method returns false.
double chartSpaceX = 1, chartSpaceY = 1;
Vetor3 worldSpace;
if(Chart.PointToWorldSpace(out worldSpace, chartSpaceX , chartSpaceY , "Player 1"))
{
// worldSpace now contains the worldspace/canvas coordinates equivalent to chart positiosn 1,1
}
2. PointToClient
gets a world space point and converts it to chart coordinates . For overlay canvas charts , it will convert from screen points.
double chartSpaceX, chartSpaceY;
Vetor3 worldSpace = new Vector3(1,1,0);
Chart.PointToClient(worldSpace ,out chartSpaceX,out chartSpaceY);
// chartSpaceX,chartSpaceY now contain the chart coordinates equivalent to worldSpace
3. TrimRect
On some uses case we would like to use rectangles instead of points, and therefore it is important to know which part of a rectangle is contained in the view portion. (check out tutorial/marker to see an example). This method take a rectangle in chart coordinates and returns a trimmed rectangle that is completely contained in the view portion. If the specified rectangle is completely out of the view portion , the method returns false.
DoubleRect untrimmedRect;
DoubleRect trimRect;
if (Chart.TrimRect(untrimmedRect, out trimRect))
{
// trimRect new contains the intersection of untrimmedRect with the view portion.
}
4. IsRectVisible
returns true if any portion of a chart coordinate rectangle is visible, returns false if the rectangle is completely off the view portion
DoubleRect rectangle;
if(Chart.IsRectVisible(rectangle))
{
// the rectangle is visible inside the view portion
}
5.RectToCanvas
On many cases we would like to display objects on top of canvas charts. These objects are also canvas object with a rect transform. This method takes a rect transform and sets it so that it will cover the specified chart coordinate rectangle. It is used in the marker tutorial in Tutorials/Marker
RectTransform trackerObject;
DoubleRect chartCoordRect;
Chart.RectToCanvas(trackerObject, chartCoordRect);
// now 'trackerObject' is placed on top of the canvas position of 'chartCoordRect'
6.MouseToClient
If we want to peak the mouse position for canvas charts , this method gets the mouse position on the graph in axis units. returns true if the mouse is in bounds of the chart , false otherwise
double mouseChartCoordX,mouseChartCoordY;
if (Chart.MouseToClient(out mouseChartCoordX, out mouseChartCoordY))
{
MouseText.text = string.Format("{0:0.00} , {1:0.00}", mouseChartCoordX, mouseChartCoordY);
//mouseChartCoordX and mouseChartCoordY contain the chart coordinates equivalent to the mouse position.
}
Bar Chart
the following method allow you to convert between chart space and world space on the Bar chart:
1. GetBarTrackPosition
Call this method to get the world space position of the top of the selected bar. The bar is specified using a category and a group. This method will return true on success or false on failure (means the category or group are non existent)
Vector topPosition;
if(bar.GetBarTrackPosition ("category","group",out topPosition))
{
//topPosition now contains the top position of the bar
}
2. GetBarBottomPosition
Same as the above method only that it returns the base position of the bar.
Vector bottomPosition;
if(bar.GetBarBottomPosition ("category","group",out bottomPosition))
{
//bottomPositionnow contains the bottom position of the bar
}
3. PointToWorldSpace
Call this method to get the world space position of a bar regardless of it’s value. This is like the above methods only that the value is specified by the call. Also set allowOverflow to true in order to specify a value which is not in the range of the chart axis
public bool PointToWorldSpace(string category, string group, double value,bool allowOverflow, out Vector3 point) |
Vector customPosition;
if(bar.PointToWorldSpace("category","group",5.0,true,out customPosition))
{
//customPosition contains the world position of value 5.0 in "category" and "group"
}
Radar Chart
the following method allow you to convert between chart space and world space on the Radar chart:
1. ItemToWorldPosition
This method takes an amount and converts it to a world position along a radar group. return true on success and false on failure (fails only if the chart was not drawn yet).
Vector3 worldPosition;
if(radar.ItemToWorldPosition("group",5.0,out worldPosition))
{
//worldPosition contains the equivalent of "group" value 5.0
}
2. SnapWorldPointToPosition
this method takes a world position and returns the radar coordinate closest to this position.The radar coordinate is specified using a group and amount. return’s true on success, false on failure (fails only if the chart was not drawn yet).
string group;
double amount;
Vector3 worldSpace;
if(radar.SnapWorldPointToPosition(worldSpace,out group,out amount))
{
// group and amount contain the radar coordinate closest to the world point.
}
Listening to CHANGES in the view portion
When the view portion is changed , it may be a good time to refresh world positions held in your own scripts. . In order to be notified about changes in the view portion , use the OnRedraw event in your chart.
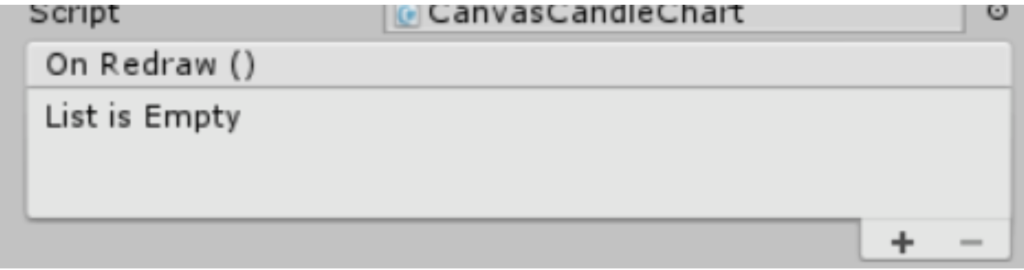
chart.OnRedraw.AddListener(myListener);
void myListener()
{
// update world positions from 'chart'
}
Getting a charts DIMENSIONS
On some occasions we would like to know the actual dimensions of a chart. The chart dimensions are available in the following properties:
var chart = GetComponent<AnyChart>();
float width = chart.TotalWidth;
float height = chart.TotalHeight;
float depth = chart.TotalDepth;
// now you can make use of the dimensions of 'chart'