We would sometimes want to have our own custom code that handles events such as user click or hover. Graph and Chart allows you to easily add your own code using Unity Events . This is a simple tutorial that will show you how it’s done. This tutorial is based on the script in Script\Utils\InfoBox . You can see it work in some scenes in Graph and Chart, for example in Themes\2d\Bar\Preset2
scroll down for the written tutorial
Creating a handler for Events
before we hook up an event , we need to create the code that handles it. In this example we will use the bar chart , but it applies to all charts , you can handle a bar chart event in the following way:
public void BarHovered(BarChart.BarEventArgs args)
{
infoText.text = string.Format("({0},{1}) : {2}", args.Category, args.Group, args.Value);
}
Notice that the method you are using has to have void as a return type , and it has to receive only one argument of type BarChart.BarEventArgs . The method also has to be public for it to show in the inspector. From within this method you can make use of the data in “args” , args contains information relevant to the specific event that has just happened.
Hooking The event
Once we have an event handler method , we need to hook it to the a chart. This way it will be called every time the event occurs. You can hook an event either from the inspector or from code:
Note: the info box events are not meant to be hooked using the inspector , this is a demonstration of how you can set up events from other scripts. Later we will see how the info box hooks events from code.
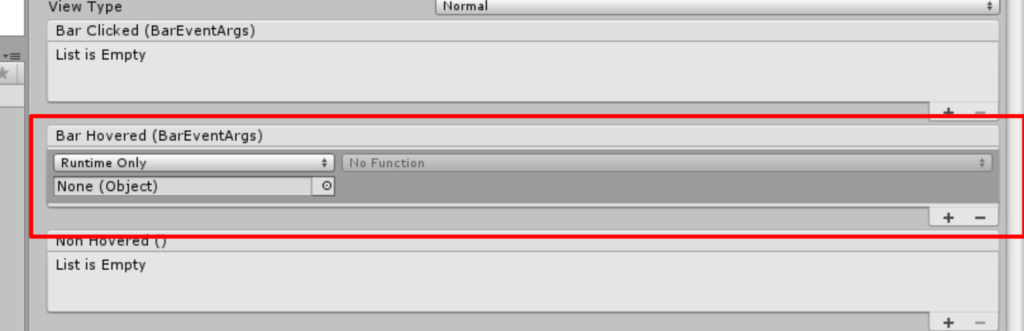
From the inspector , you can locate the event on the chart object , then drag and drop the handler object :
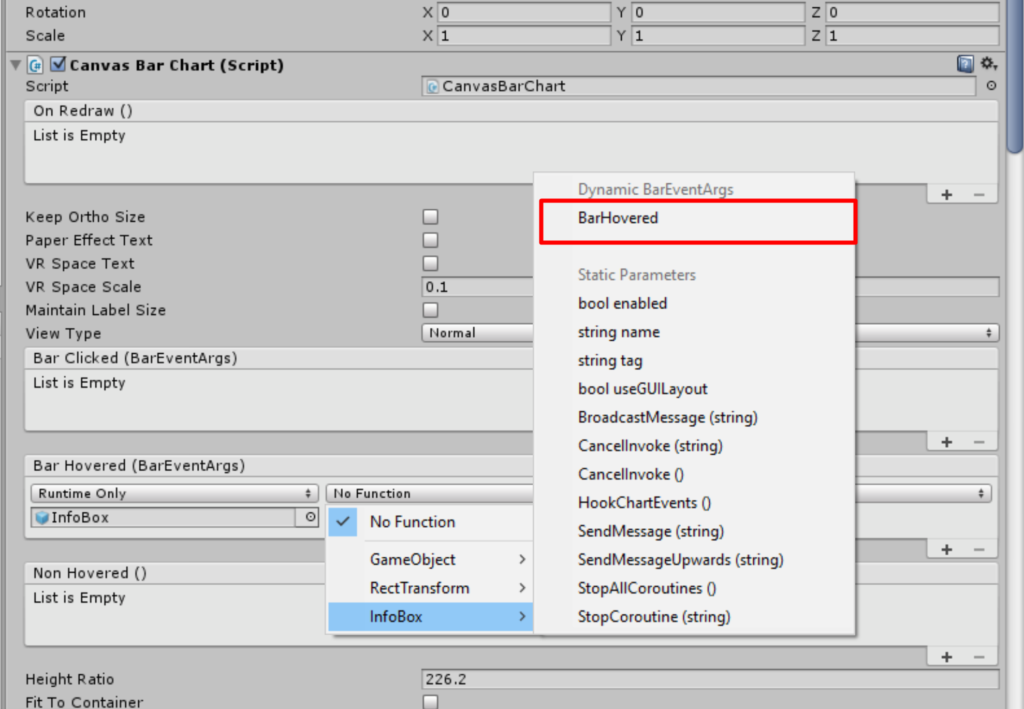
Now with some use cases we would like to hook the event from code. This can be done like this :
bar.BarHovered.AddListener(BarHovered);
once this method is called you will have your method called each time the event happens.
Chart Leave event
After the user is done hovering over the chart , we would like to clear the text box we have filled in the method BarHovered. for this purpose there is an important event called NonHovered. This event is called once there is not longer an interaction with the chart. here is an example of how you can set it :
bar.NonHovered.AddListener(NonHovered); // call this method to hook the event listener
...
void NonHovered()
{
infoText.text = ""; // clear the textbox
}
How to make different interactions for different chart objects
A lot of times we would like to make different interactions for different chart items . For example, we would like to load a different UI element whenever a bar is clicked. You can distinguish between bars using the parameters in the event args. Each bar is defined by it’s Group and Category. So we can load UI based on the Group and Category provided in the event args. For graph chart , you can use the category and the index provided in the event args.
Event args reference
in this section we will go through the event args classes and their properties
1. BarEventArgs

- TopPosition is the position of the top part of the bar item. The position is in world space or in canvas coordinates depending on the type of chart
- Value is the value or amount set for the bar item.
- Category is the category to which the bar belongs to
- Group is the group to which the bar belongs to
2. GraphEventArgs
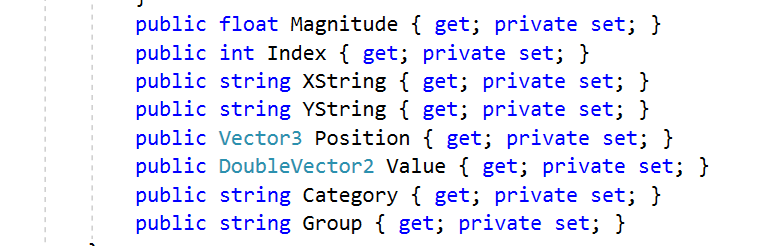
- Magnitude is the size of the point for a bubble chart or a size based graph
- Index is the index of the graph point that recived the event. This index is into the data array of the graph category
- XString/YString are human readable strings that portray the x and y values according to the graph chart’s settings
- Position is the position where the event took place. It is either in world space coordinates or canvas coordiantes depending on the type of graph chart.
- Value is the value of the point that is interacted with
- Category is the category of the point that is interacted with
- Group is not used and is reserved for future use
3. PieEventArgs

- Value is the value of the pie item that was interacted with.
- Category is the category of the pie item that was interacted with.
4.RadarEventArgs
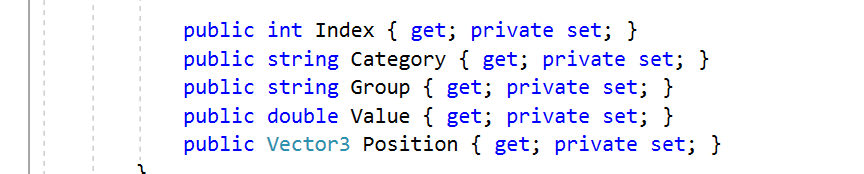
- Index is the index of the item that was interacted with
- Category is the category of the item that was interacted with
- Group is the group of the item that was interacted with
- Value is the value of the item that was interacted with
- Position is the position where the interaction took place. This is the positions in world space coordinates or in canvas coordinates depending on the type of radar chart being used
5. CandleEventArgs
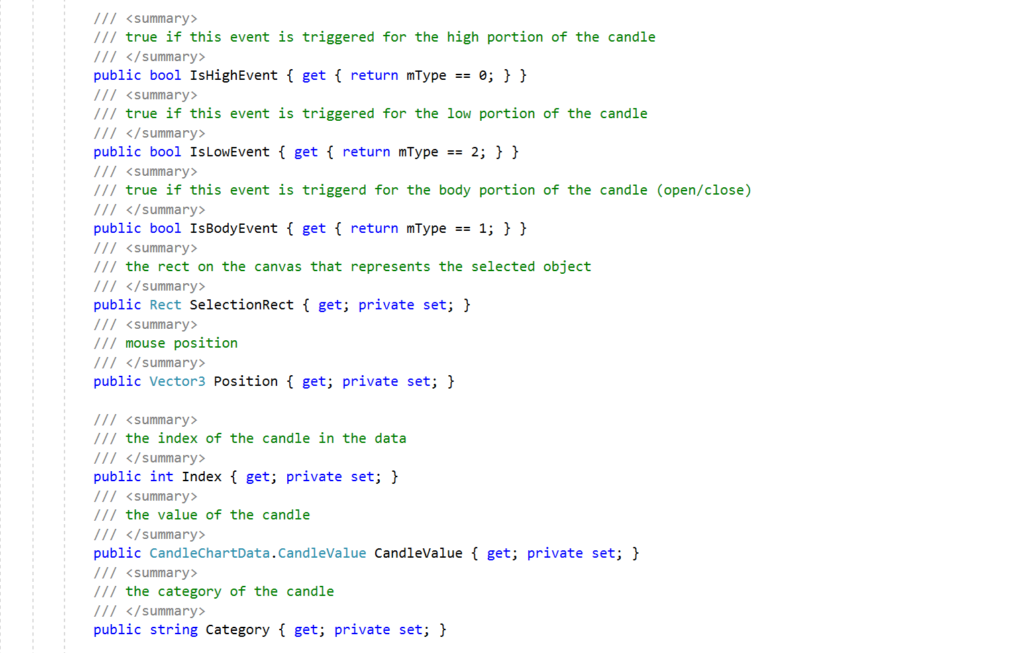