This article describes the methods that manipulate data on the radar chart. You can also have a look at the Radar Chart Quick Start Tutorial
HOW TO QUICKLY SET THE Radar CHART DATA USING THE INSPECTOR
When creating a radar chart. You can quickly set it’s values in the inspector by click “Edit Values” in the data section of the radar chart component:
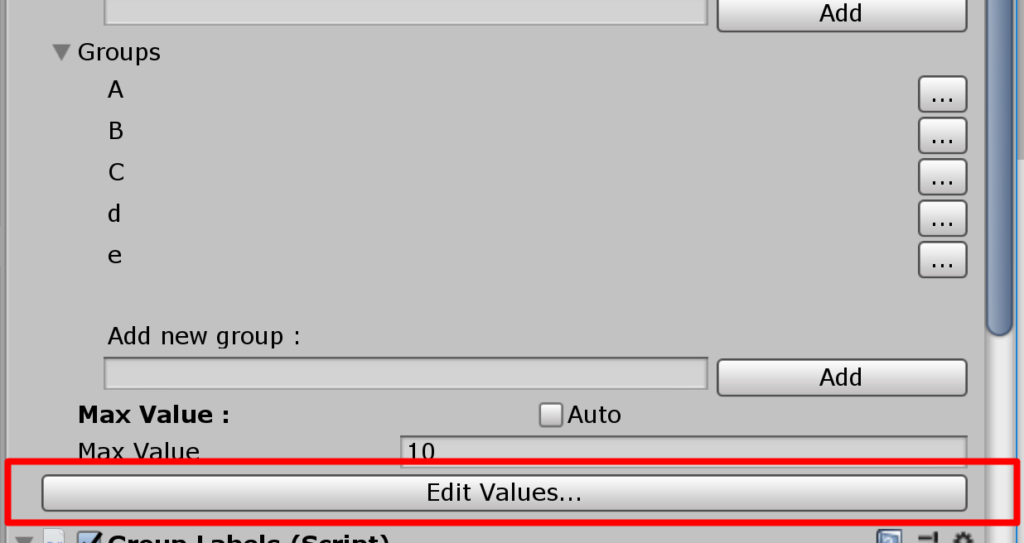
Once you click on it , you will see the edit values window :
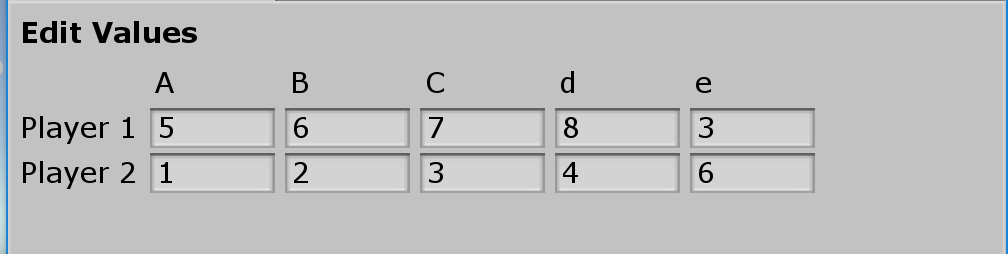
Each cell corresponds to a category and group. Any value change will immediately reflect in the scene view.
How to set the values of the radar chart
You can set the values of the radar chart by calling SetValue
double RadarValue = 5;
radar.DataSource.SetValue("Category", "Group", RadarValue);
USE CASE EXAMPLE
Let’s say you have an sql data query and would like to apply it’s first row to the radar chart . The row is made of columns with values each matches a radar group. The radar chart has a category named “firstRow” that was previously configured in the inspector. Make sure to view Configuring groups and categories from script to learn how to add groups to the radar chart. Here is a possible way you go about applying the data row to the chart:
SqlDataReader reader = command.ExecuteReader();
var radarChart = GetComponent<RadarChart>();
// clear the groups before adding new ones
radarChart.ClearGroups();
// add the columns from the data table as radar groups
for(int i=0; i<reader.FieldCount; i++)
{
// Add the group to the radar chart
// The group is named after the column in the data row
radarChart.DataSource.AddGroup(reader.GetName(i));
}
// read the first line only
if(reader.Read())
{
for(int i=0;i<reader.FieldCount;i++)
{
// get the value of each column
double groupValue = reader.GetDouble(i);
// apply the value to the radar chart. The group name being the column name
radarChart.DataSource.SetValue("firstRow",reader.GetName(i), groupValue);
}
}
reader.Close()
As you can see we are making using use ADO .NET in order to bring data from an sql table. We take the name of each column and create a group by the same name. We then read the first row and set the values for each group in “firstRow”.