The resulting scene and script from this tutorial can be found at Assets\Chart and Graph\InBeta\Tutorials\Candle
Go to InBeta\Themes\2D
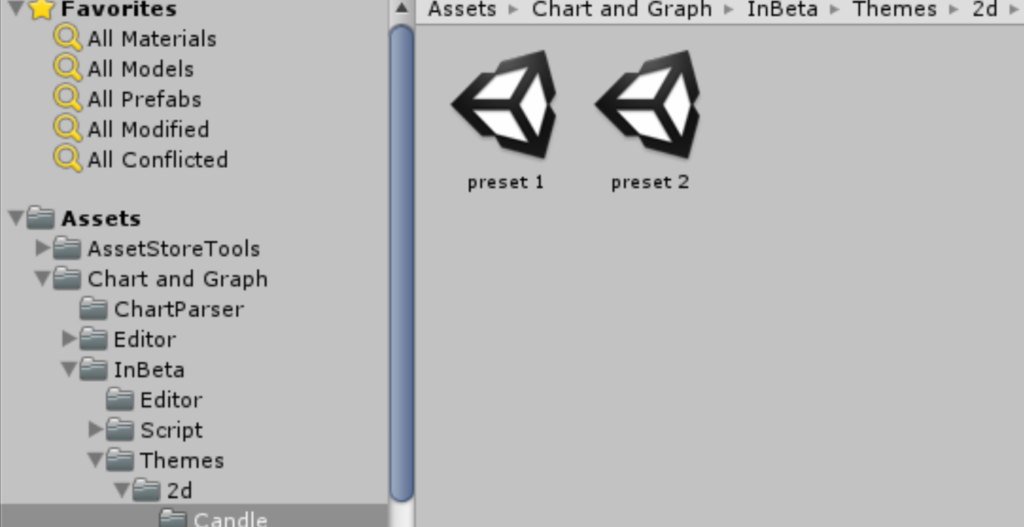
Select a preset scene and duplicate it. We will use it as a foundation for this tutorial.
In the duplicated scene , go to the candle chart object and select it. Go to the data section in the inspector :

Expand the categoies. You can add categories by typing a name and clicking the add button. You can remove and rename categories by clicking the “…” button near each one. For the sake of demonstration , We will assume that you have one category named “Player 1”. the data section of your inspector should look like the image above.
To update the candle chart from your script , create a new monobehaviour named CandleChartFeed and add the following code to it:
void Start()
{
CandleChart candle = GetComponent<CandleChart>();
if (candle != null)
{
candle.DataSource.StartBatch();
candle.DataSource.ClearCategory("Player 1");
for (int i = 0; i < 30; i++)
{
candle.DataSource.AddCandleToCategory("Player 1", new CandleChartData.CandleValue(Random.Range(5f, 10f), Random.Range(10f, 15f), Random.Range(0f, 5f), Random.Range(5f, 10f), i * 10f / 30f, Random.value * 10f / 30f));
}
candle.DataSource.EndBatch();
}
}
As you can see , you can add candles by calling AddCandleToCategory. You can create a candle value with ohlc values . This example generates random candles.
Add a CandleChartFeed component to the candle chart object and click play.
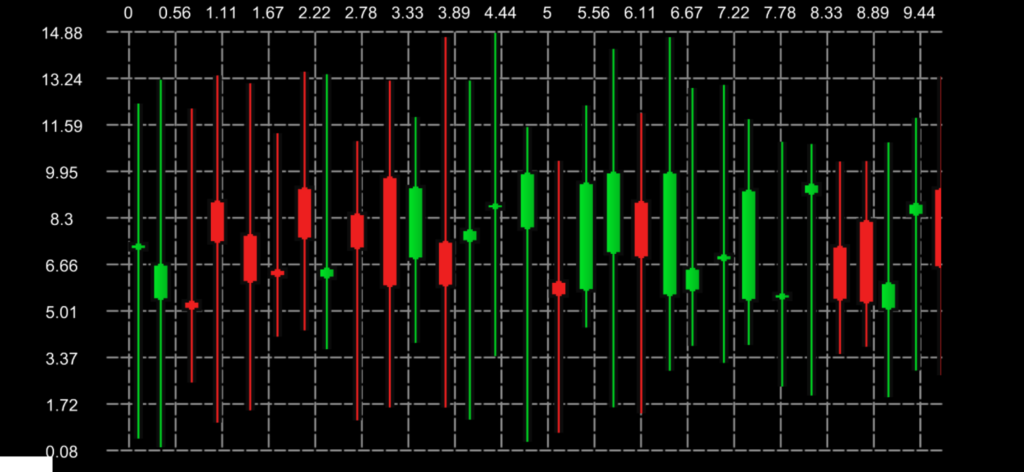
Add streaming candles
You can call AddCandleToCategory from your update method in order to stream new data to the chart. Note that the time of the new candle has to be after the latest one currently in the chart. Add the following code to your script :
float time = 3f; //
float x = 10f;
private void Update()
{
time -= Time.deltaTime;
if (time <= 0)
{
time = 3f;
x += 0.3f;
CandleChart candle = GetComponent<CandleChart>();
candle.DataSource.AddCandleToCategory("Player 1", new CandleChartData.CandleValue(Random.Range(5f, 10f), Random.Range(10f, 15f), Random.Range(0f, 5f), Random.Range(5f, 10f), x, Random.value * 10f / 30f), 1f);
}
}
The script we have created times the insertion of new random candles. Notice that we can pass animation time to AddCandleToCategory .
Click play , you will now have a streaming candle chart